Rust Continue Statement
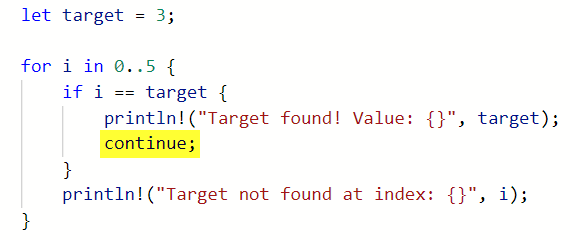
In the Rust programming language, the continue statement is used to skip the rest of a loop iteration and jump to the next iteration.
The continue keyword is used when you want to stop the current iteration of a loop and start the loop at the next iteration. If you want to terminate the loop, use a break statement instead.
Continue is often placed within a conditional expression (like an if…else statement) so that the loop iteration stops when a specific condition is met.
Continue statements can be used with for, loop, and while loops. We will cover examples of using continue with each type of loop below.
Using continue in a for loop
The continue statement can be used in a for loop. The following example uses a for loop to determine the value of a target:
let target = 3;
for i in 0..5 {
if i == target {
println!("Target found! Value: {}", target);
continue;
}
println!("Target not found at index: {}", i);
}
Standard Output:
Target not found at index: 0
Target not found at index: 1
Target not found at index: 2
Target found! Value: 3
Target not found at index: 4
The continue keyword is used here to identify the target and prevent iteration of the rest of the for loop. Note that the for loop continues to run until it reaches the maximum value specified in the range declaration (0..5).
Using continue with a loop
The loop keyword creates an infinite loop, and continue can be used to stop a single iteration. Note that when using the loop keyword, continue isn’t sufficient to break out of the loop; for that, we need to use break.
let target1 = 5;
let target2 = 7;
let mut i = 0;
loop {
i += 1;
if i == target1 {
println!("Value of target 1: {}", target1);
continue;
}
else if i == target2 {
println!("Value of target 2: {}", target2);
break;
}
}
Standard Output:
Value of target 1: 5
Value of target 2: 7
When the loop reaches the continue statement, the loop restarts. The break statement is needed to prevent it from running infinitely. The combination of the continue and break statements provides us with options to support control flow.
Using continue in a while loop
The while loop only executes when the specified condition is true.
As with the other loop types, continue is used to integrate control flow functionality by restarting the loop.
In the following example, we are using a while loop to limit our scan to 255. Within the loop, we use the continue statement to exit the while loop when the target is identified.
let target1 = 5;
let target2 = 7;
let mut i = 0;
while i <= 255 {
if i == target1 {
println!("Value of target 1: {}", i);
i += 1;
continue;
}
else if i == target2 {
println!("Value of target 2: {}", i);
break;
}
else {
println!("Loop index: {}", i);
i += 1;
}
}
Standard Output:
Loop index: 0
Loop index: 1
Loop index: 2
Loop index: 3
Loop index: 4
Value of target 1: 5
Loop index: 6
Value of target 2: 7
Note that the continue statement allows the loop to continue running. It allows us to execute other code based on conditionals without exiting the loop.
What Happens if You Don’t Use a continue Statement?
In many cases, we can simply choose not use a continue statement. If we don’t use a continue statement, then the remaining code in the loop body will be evaluated.
While it’s easy to structure code in a way that such a loop would function, there are many cases when we can optimize code using the continue statement. This is because the continue statement prevents evaluation of the rest of the code.
Consider the example we saw earlier:
let target = 3;
for i in 0..5 {
if i == target {
println!("Target found! Value: {}", target);
continue;
}
println!("Target not found at index: {}", i);
}
Standard Output:
Target not found at index: 0
Target not found at index: 1
Target not found at index: 2
Target found! Value: 3
Target not found at index: 4
In this case, the continue statement is used to prevent the println! macro from printing the line “Target not found at index: 3”. Thus it makes the code more efficient and it promotes better functionality.
Note that there is some sloppiness in this example: this code would be more explicit if we used an else statement instead of just printing the line below the if statement.