Rust Floating Point Numbers
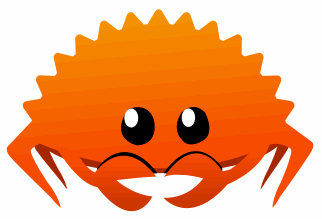
Floating point numbers are numbers that have a decimal point.
In Rust, floating point numbers are represented by the f32
and f64
types. An f32 is a 32-bit float, and an f64 is a 64-bit float. These are sometimes known as single-precision and double-precision floating point numbers, respectively.
The f32 and f64 types are defined in the std::f32
and std::f64
modules of the standard library.
Floating point numbers are useful for representing decimal values that cannot be accurately represented using integers. They are often used in scientific and engineering applications, where precise decimal values are required.
Using Floats in Rust
To use floating point numbers in Rust, we can declare a variable using the f32
or f64
type, depending on the precision required. For example:
let x: f32 = 3.14159;
let y: f64 = 2.718281828459045
We can perform various mathematical operations on floating point numbers, such as addition, subtraction, multiplication, and division.
The following example shows how to perform basic arithmetic using floats:
let a = 3.0;let b = 2.5;
let c = a + b; // 5.5
let d = a - b; // 0.5
let e = a * b; // 7.5
let f = a / b; // 1.2
Rust Floating Point Number Rounding Errors
It’s important to keep in mind that floating point numbers are not always precise, due to the way they are represented in memory.
As a result, you may see some rounding errors when working with floating point numbers in Rust. For example:
let x = 0.1 + 0.2; // 0.30000000000000004
Overall, floating point numbers are a useful data type in Rust and many other programming languages. They allow us to work with decimal values, but we need to be aware of their potential imprecision when working with them.
Converting an Integer to a Float in Rust
We can convert an integer to a floating point number in the Rust programming language. To do so, we can use the as
keyword followed by the type you want to convert the integer to.
For example, to convert an i32
integer to an f64
floating point number, we can use the following syntax:
let x: i32 = 5;
let y: f64 = x as f64;
Alternatively, we can use one of the conversion methods provided by the std::convert
module. For example, we can use the from
method to convert an i32
integer to an f64
floating point number like this:
let x: i32 = 5;
let y: f64 = f64::from(x);
We can also use the into
method, which is a bit more concise:
let x: i32 = 5;
let y: f64 = x.into();
These methods are more flexible and can be used to convert between a wider range of types. They also allow you to specify additional conversion options, such as the desired precision or rounding behavior.
Overall, there are several ways to convert an integer to a floating point number in Rust. We can use the as
keyword, the from
method, or the into
method, depending on your needs and preferences.