Rust Match Expression
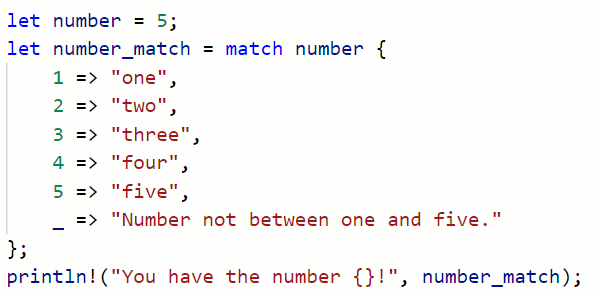
In Rust, match is a type of conditional expression that checks to see if a value corresponds with any value on a list of values.
Match expressions are great because they simplify code when compared with a long list of if/else statements. In other words, the match keyword allows a simpler syntax than if/else statements, which is useful when there are many values that we want to be evaluated for comparison.
In this article, we will cover match statement overview and syntax, assigning the output of a match expression to a variable, and the differences between if expressions and match expressions.
What are Match Expressions?
Match expressions are a type of conditional expression. While if expressions will run code based on the truthiness of an expression, match runs code based on whether one value is equal to another value.
Match statements use the match keyword. In the following example, match_this is the value that val_1, val_2, and val_3 are matched against. If any of the values are equal to match_this, the corresponding code block will be executed:
match match_this {
val_1 => {
// This code block executes if match_this equals val_1
},
val_2 => {
// This code block executes if match_this equals val_2
},
val_3 => {
// This code block executes if match_this equals val_3
},
_ => {
// This block executes if the others don't.
}
};
Rust forces us to include a default statement, which will execute if no match is found. The default statement is specified using an underscore ‘_‘.
The following code demonstrates a simple example of using a match statement to print out different statements depending on the value of the number variable:
let number = 5;
match number {
1 => println!("You have one!"),
2 => println!("You have two!"),
3 => println!("You have three!"),
4 => println!("You have four!"),
5 => println!("You have five!"),
_ => println!("Number not between one and five."),
};
Standard Output:
You have five!
Match Catch-All
Rust allows us to include a catch-all statement that executes if none of the other statements provide a match. The previous examples included a catch-all at the end using an underscore:
_ => {expression}
This is similar to using an else statement at the end of an if…else expression. Technically, the catch-all can be any valid variable name; it doesn’t need to be an underscore. However an underscore is commonly used because Rust won’t throw an error for an unused variable that starts with an underscore.
Assign Match Result to a Variable
The result of a match expression can be assigned to a variable so that it can be retrieved for use later in the code.
One restriction on this method is that the output of the match statement must be a value that can be stored inside a variable. We can’t use it to call a macro such as println!().
To assign the result of a match expression, we can use the let keyword as if we were declaring a variable of any other type:
let my_variable = match match_this {
val_1 => {
},
val_2 => {
},
val_3 => {
},
_ => {}
}
Let’s see how we can apply this in our number matching example:
let number = 4;
let number_match = match number {
1 => "one",
2 => "two",
3 => "three",
4 => "four",
5 => "five",
_ => "Number not between one and five."
};
println!("You have the number {}!", number_match);
Standard Output:
You have the number four!
Note that in this case, the matching condition results in an output that is equal to a string value. If we tried to print a statement directly in the match expression, our code wouldn’t have compiled correctly.
Match vs. If Expressions in Rust
Match and if statements can be used to accomplish similar tasks but there are some key differences:
if expression | match expression |
Evaluates a Boolean statement for truthiness | Evaluates a pattern to be matched |
Ideal for small number of conditions | Cleaner code for larger number of conditions |
Can evaluate a range of conditions | Value only – condition cannot take range |
From this table, we can see that there are use cases when if expressions make more sense to use than match expressions. However in many cases, a match expression is the best choice when we want to perform simple conditional matching for multiple possible cases.