Rust Assignment Operators
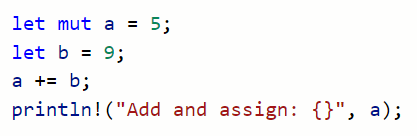
In the Rust programming language, an assignment operator is used to assign a value to a variable.
Variables can be assigned a value using the assignment operator ‘=’:
fn main() {
let a = 5; // a is being assigned a value of '5'
println!("Value of 'a': {}", a);
}
Standard Output:
Value of 'a': 5
The assignment operator is represented by the equal sign ‘=‘. In this example, it is used to assign an integer value of 5 to the variable a.
Variables can also be assigned a new value using the assignment operator:
fn main() {
let mut a = 5; // a is assigned an initial value
println!("Initial value of 'a': {}", a);
a = 13; // a is assigned a new value
println!("New value of 'a': {}", a);
}
Standard Output:
Initial value of 'a': 5 New value of 'a': 13
The variable needs to be mutable in order for us to assign a new value to it. This can be done using the ‘mut’ keyword.
Types of Assignment Operators
We’ve seen how the assignment operator can be used to assign an initial value to a variable, as well as change its value after the initial assignment. In addition to the simple assignment operator, there are several other types of assignment operators, all of which are compound assignment operators.
A compound assignment operator performs some type of action in addition to assignment. For example, we can add and assign using the ‘+=’ operator or subtract and assign using the ‘-=’ operator.
The following table covers the types of assignment operators and their functionality. The first is the simple assignment operator and the rest are all compound assignment operators.
Table of Assignment Operators in Rust
Operator | Function | Syntax |
= | Assign | a = b |
+= | Add and assign | a += b |
-= | Subtract and assign | a -= b |
*= | Multiply and assign | a *= b |
/= | Divide and assign | a /= b |
%= | Modulus and assign | a %= b |
&= | Bitwise AND and assign | a &= b |
|= | Bitwise OR and assign | a |= b |
^= | Bitwise XOR and assign | a ^= b |
<<= | Bitwise Left Shift and assign | a <<= b |
>>= | Bitwise Right Shift and assign | a >>= b |
Compound Assignment Operators in Rust
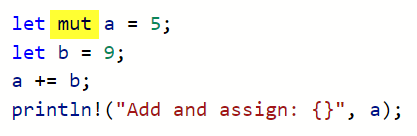
There are ten (10) compound assignment operators in Rust. These allow the code to be a little simpler by combining two operations.
Compound assignment operators are only used after the variable binding and assignment, and they change the value of the initial assignment. In order to change the value of a variable in Rust, it must be made mutable using the mut keyword.
The following example shows how to use the compound assignment operators:
fn main() {
let mut a = 5; // Initial assignment, includes 'mut'
println!("Initial value of 'a': {}", a);
a += 8;
println!("New value of 'a': {}", a);
}
Standard Output:
Initial value of 'a': 5
New value of 'a': 13
Of the compound assignment operators, 5 are used for arithmetic operations, and the other 5 perform bitwise operations. You can learn more about each individual operator in the pages that cover these two operation types.