Printing in Rust
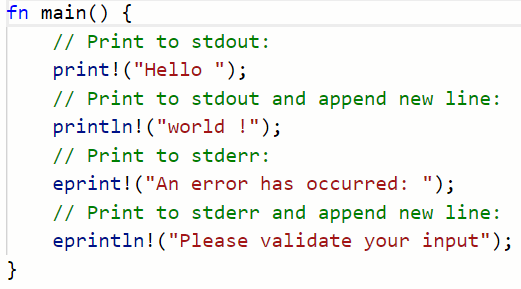
The Rust programming language includes four macros that can be used to print: print!(), println!(), eprint!(), and eprintln!().
The first two print to the standard output ‘stdout‘, while the last two print to standard error ‘stderr‘.
The functionality of each macro can be found in the following table:
Macro | Functionality | Prints to |
print!() | Prints string to standard output | stdout |
println!() | Prints string to standard output and appends new line | stdout |
eprint!() | Prints output to standard error | stderr |
eprintln!() | Prints output to standard error and appends new line | stderr |
In general, the println!() macro is most commonly used for basic console output functionality because it prints and also appends a new line. This leads to much more readable output, in a format that coders tend to expect (rather than printing multiple output strings on the same line).
In this article, we’ll cover the usage of these four macros that allow us to print to the console in Rust.
The print!() Macro
The print!() macro is used to print text to the standard output (usually the console).
Here is an example of how we can use the print!() macro:
print!("Hello, ");
print!("world!");
This code will print Hello, world!
to the standard output. The “Hello, world!” string is printed on a single line because print!() does not append a new line.
This is the most basic printing operation in Rust, but is less popular when compared with the println!() macro.
The println!() Macro
The println!() macro does two things:
- It prints to the standard output.
- It appends a new line at the end of the printed string.
This makes the output much more readable. We can use the println!() macro in virtually the same way as the print!() macro:
println!("Hello, world!");
println!("I love Rust!");
Output:
Hello, world!
I love Rust!
Note that in this case, “Hello, world!” is printed on one line, and “I love Rust!” is printed on the next line because the println!() macro automatically created a new line.
The eprint!() Macro
In Rust, the eprint!() macro is similar to the print!()
macro, but it prints to the standard error 'stderr'
instead of the standard output ‘stdout'
.
In other words, the output is actually sent to a different destination, which can be useful in many situations. The standard error is typically used for printing error messages or other diagnostic information, so that it can be easily distinguished from the regular output of the program.
Here is an example of how we can use eprint!():
eprint!("An error has occurred: ");
eprint!("invalid input\n");
This code will print “An error has occurred: invalid input
” to the standard error, followed by a newline.
You can use the eprintln!() macro to print to the standard error and add a newline at the end, in the same way as the println!()
macro works for the standard output.
The eprintln!() Macro
Similar to the functionality difference between print!() and println!(), the eprintln!() macro adds to eprint!() by automatically appending a new line to the end of the string.
eprintln!() prints to stderr instead of stdout, making it ideal for working with error messages. However, the inclusion of a new line tends to make eprintln!() more popular, as developers don’t need to manually include a new line character at the end of the string.
eprintln!("An error has occurred.");
eprintln!("Please validate your input.");
Output:
An error has occurred.
Please validate your input
Standard Output vs. Standard Error in Rust
In Rust, standard output (stdout
) and standard error (stderr
) are two streams where a program can write output. Standard output is used for regular program output, while standard error is used for diagnostic information and error messages.
The main difference between the standard output and standard error is that they are typically handled differently by the operating system.
For example, standard output may be sent to the console, while the standard error may be sent to a different destination, like a log file or a different terminal window. This allows the regular output of the program to be easily distinguished from error messages and other diagnostic information.
Printing in Rust – Conclusion
In this article, we’ve covered four primary macros used for printing in the Rust programming language.
Two of them (print!() and println!()) are used to print to the standard output (stdout), while the other two (eprint!() and eprintln!()) are useful for printing errors to the standard error (stderr). We also dove into the details of standard output vs standard error in Rust.
In addition to printing the, println!() and eprintln!() macros append a new line to the end of the string being printed. This is a highly useful feature which tends to make them appealing, since developers don’t need to manually include a new line character in the string.