Rust loop
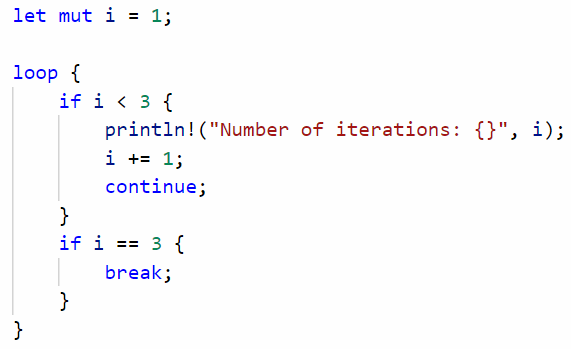
In Rust, the loop keyword is used to define an infinite loop. The code block defined by loop will execute repeatedly until the break keyword is used.
loop iterates for a number of times that is unknown at compile time, making it an indefinite loop. Instead of iterating for a known number of times (like a for loop), loop iterates until a condition is met.
The basic syntax uses the keyword loop:
loop {}
The code inside the curly braces ‘{}’ will be looped over. For example:
loop {
println!("This is a loop");
}
This creates an infinite loop that prints “This is a loop” until the program is terminated.
Using the ‘break’ keyword
The break keyword is used to terminate a loop.
If we just use break inside the body of the loop, then the loop will terminate the first time that the code executes:
loop {
println!("This is a loop");
break;
}
Standard Output:
This is a loop
This is of limited value, because the whole point of a loop is to iterate multiple times.
In order to create a useful loop, break is often located inside a conditional expression such as an if statement:
let mut i = 0;
loop {
println!("{}",i);
if i == 3 {
break;
}
i += 1;
}
Standard Output:
0
1
2
3
In this example, we create a mutable variable named i. Inside the loop, we print the value of i and then increment its value. Next, we use an if expression to check to see if i is equal to 3. When i equals 3, the if expression executes, triggering break.
The value of i starts at 0 with the first loop. The loop repeats and i gets incremented by 1 until the third loop. On the fourth loop, i gets printed to the terminal but the if expression executes and triggers break, stopping the loop execution at that point.
Using continue
The continue keyword can be used to skip the rest of the current loop and start the loop again from the beginning.
Unlike break, continue will not exit the loop entirely.
let mut i = 1;
loop {
if i < 3 {
println!("Number of iterations: {}", i);
i += 1;
continue;
}
if i == 3 {
break;
}
}
Standard Output:
Number of iterations: 1
Number of iterations: 2