Rust Loop Nesting
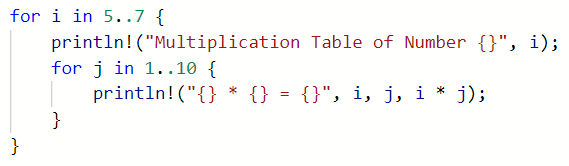
In Rust, loops can be nested inside of each other, allowing the creation of a loop inside of another loop. This gives us more options for improved control flow.
Loops are nested by placing one loop inside the code block of another loop. The code block of each loop is defined by curly braces {}.
loop {
// Body of outer loop
loop {
// Body of inner loop
}
}
Note that any kind of loop can be nested in any other kind of loop. For example, we can nest a for loop inside of a while loop or an infinite loop.
In the following example, we are using nested for loops to print out multiplication tables:
for i in 5..7 {
println!("Multiplication Table of Number {}", i);
for j in 1..10 {
println!("{} * {} = {}", i, j, i * j);
}
}
Standard Output:
Multiplication Table of Number 5
5 * 1 = 5
5 * 2 = 10
5 * 3 = 15
5 * 4 = 20
5 * 5 = 25
5 * 6 = 30
5 * 7 = 35
5 * 8 = 40
5 * 9 = 45
Multiplication Table of Number 6
6 * 1 = 6
6 * 2 = 12
6 * 3 = 18
6 * 4 = 24
6 * 5 = 30
6 * 6 = 36
6 * 7 = 42
6 * 8 = 48
6 * 9 = 54
When we use nested loops, the outer loop executes until the inner loop is reached. Then, the inner loop executes repeatedly until it terminates. The outer loop is then executed again, triggering the inner loop again. This occurs until the outer loop is terminated.