Iterating Over a Vector
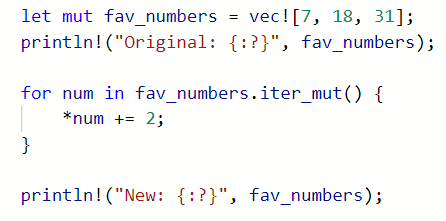
In Rust, there are several ways to iterate over the elements of a vector. The most common methods are using a for loop, or using the iter() method.
Iterating Over a Vector Using a for Loop
We can use a for loop to iterate over each element in a vector:
let fav_colors = vec!["green", "blue", "red"];
for color in fav_colors {
println!("My favorite colors are: {}", i);
}
Standard Output:
My favorite colors are: green
My favorite colors are: blue
My favorite colors are: red
Using the .iter() Method
The .iter() method can be used to iterate over a vector with or without a for loop. When used with a for loop, we can call the .iter() method following the name of the vector:
let fav_colors = vec!["green", "blue", "red"];
for color in fav_colors.iter() {
println!("My favorite colors are: {}", i);
}
Standard Output:
My favorite colors are: green
My favorite colors are: blue
My favorite colors are: red
As we can see, the .iter() method doesn’t need to be called when using a for loop. However if we want to change the vector, we can do this using a similar method called .iter_mut().
Changing Vector Elements Using the .iter_mut() Method
We can iterate over a mutable vector and change the value of its’ elements using the .iter_mut() method.
In the body of the for loop, we need to dereference the left hand side where the vector element gets referenced. We can dereference using an asterisk ‘*‘.
In the following example, we are using the .iter_mut() method in the for loop and then dereferencing num (i.e. *num) so that we can increase the value of each vector element by 2:
let mut fav_numbers = vec![7, 18, 31];
println!("Original: {:?}", fav_numbers);
for num in fav_numbers.iter_mut() {
*num += 2;
}
println!("New: {:?}", fav_numbers);
Standard Output:
Original: [7, 18, 31]
New: [9, 20, 33]