Rust Constants
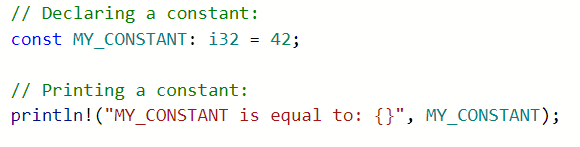
In Rust, constants are values that are immutably paired with a name. Constants cannot be changed after they have been assigned a value.
Rust uses the const keyword to declare a constant:
const MY_CONSTANT: u32 = 42;
Let’s break this down:
const is used to declare the constant.
MY_CONSTANT is the name of the constant, which uses SCREAMING_SNAKE_CASE.
u32 is the data type of the constant, in this case a 32 bit unsigned integer.
42 is the value being assigned to MY_CONSTANT
In summary, we are declaring a new constant called ‘MY_CONSTANT‘. It is of type u32, which is an unsigned 32-bit integer. Finally, we set MY_CONSTANT equal to the value ’42’.
Constants vs. Variables in Rust
There are two kinds of name-binding declarations in Rust: constant declarations and variable declarations.
Variables can be either mutable or immutable, but constants are always immutable.
We’ve seen that constants are declared using the const keyword. Variables, on the other hand, are declared using the keyword let.
Here are some of the main differences between constants and variables in Rust:
- Constants cannot be made mutable, and cannot use the mut keyword.
- Constants are declared using const, while variables are declared using let.
- Constants require the data type to be specified, while variable do not.
- Constants can be declared in any scope (including global); variables cannot.
- Constants should use SCREAMING_SNAKE_CASE; variables should use snake_case.
Declaration Keyword | Syntax | Type | |
Constants | const | SCREAMING_SNAKE_CASE | Required |
Variables | let | snake_case | Not required |
Constants vs. Immutable Variables
As we mentioned, in Rust, constants are not simply immutable variables.
Instead, the two are differentiated at a deep level to give developers more options and encourage safer coding practices.
Variables are actually immutable by default and must be made mutable by including the mut keyword when they are defined:
let mut my_variable = 42;
Naming Convention for Constants in Rust
Rust uses SCREAMING_SNAKE_CASE for constants. This means that all letters are capitalized and words are separated with an underscore
In contrast, variables use snake_case.
Compiler Errors with Rust
If an entity is declared to be a constant but its value is changed after being assigned, the compiler will throw an error.
Rust wants us to think carefully about what we are using our constants and variables for. If the value needs to be changed, then we should be using a mutable variable rather than a constant. However this also limits the scope, which encourages us to be safer.