Rust Variable Scope
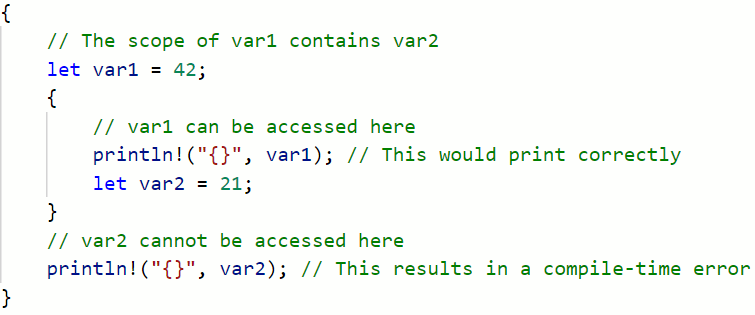
In the Rust programming language, scope refers to the part of the code over which a variable is valid and can be accessed. Scope is determined by the location of the variable declaration, and variables can’t be accessed outside of their scope.
Scope can be either global or local. Global scope refers to a variable or constant that can be accessed anywhere else in the code. Rust does not allow variables to be global, but it does allow for global constants.
Non-constant variables are limited to a local scope in Rust, which means that they must be declared within a block of code encapsulated by curly braces {}. The variable will not be accessible outside of the curly braces (i.e. outside of its’ scope).
What is Scope in Rust?
Scope in Rust is defined by location. Code blocks can be specified simply by using curly braces in the code:
fn main() {
// Create a code block:
{
let my_var = 42;
}
}
The scope of my_var is limited to the code block. If we try to call it outside of the code block, we will get a compiler error:
fn main() {
{
let my_var = 42;
}
println!("{}",my_var);
}
Standard Error (stderr):
error: cannot find value `my_var` in this scope
However, the variable can be called from anywhere inside the scope of the code block in which it is declared:
fn main() {
{
let my_var = 42;
println!("{}", my_var);
}
}
Standard Output (stdout):
42
The scope of the variable includes nested code blocks:
fn main() {
{
let my_var = 42;
{
// The println!() macro is called within the scope of my_var:
println!("{}", my_var);
}
}
}
Standard Output (stdout):
42
How to Use a Variable Outside Its Scope in Rust
You can’t use a variable outside its’ scope in Rust. Trying to use a variable outside of its scope will always result in a compile-time error. However, there are a few possible solutions available if you need a workaround:
- Use the
move
keyword to move the variable to a new scope. This will allow you to use the variable outside of its original scope. Note that it also invalidates the original variable so that it cannot be used again. - Use the
ref
keyword to create a reference to the variable. This will allow you to use the variable outside of its original scope, but it will not move the variable or invalidate the original. - Use the
Box
type to create a heap-allocated value that can be shared across several scopes. This will allow you to use the variable outside of its original scope, and it will also ensure that the variable remains valid and accessible.
It’s a good idea to use the smallest possible scope for a variable. This helps to avoid potential errors or bugs.