Rust Variable Shadowing
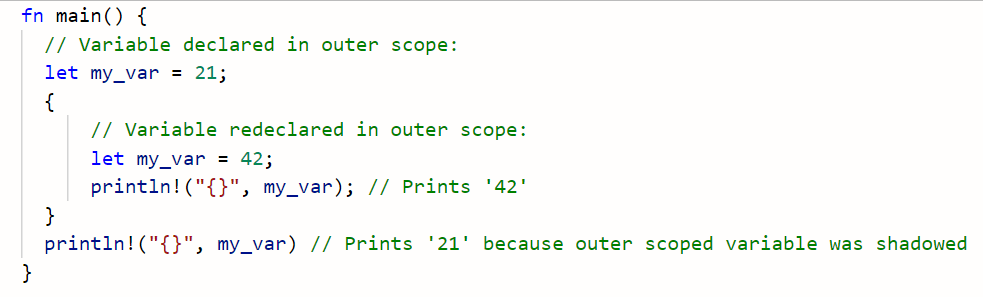
In the Rust programming language, shadowing is a technique that allows a variable to be redefined within a limited scope. Shadowing is also commonly known as masking or name masking.
Shadowing is closely related to the top of variable scope. It occurs between an ‘inner scoped’ variable and an ‘outer scoped’ variable with the same name.
When one variable is declared within the inner scope of a variable of the same name, the inner scoped variable will shadow the outer scoped variable. This means that it can take one a different value without changing the value of the value of the outer scoped variable.
In other words, shadowing refers to the fact that the inner variable prevents the outer variable from being altered in value.
Rust Scope and Shadowing
The concept of shadowing is closely related to scope. Take for example, an inner and outer scope defined by a code block ‘{}’:
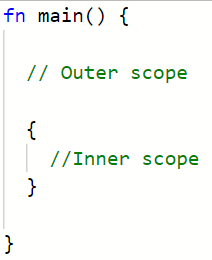
The code block defined by curly braces ‘{}’ is encompassed by the main() function, so it has a local, or inner scope.
We can declare a variable in either scope. If we use the same variable name, then the inner scoped variable will shadow the outer scoped variable:
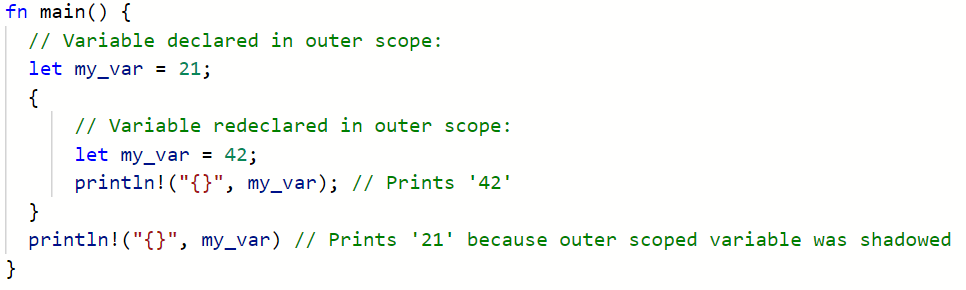
fn main() {
// Variable declared in outer scope:
let my_var = 21;
{
// Variable redeclared in outer scope:
let my_var = 42;
println!("{}", my_var); // Prints '42'
}
println!("{}", my_var) // Prints '21' because outer scoped variable was shadowed
}
In the previous example, note that the value of the outer scoped variable doesn’t change even though the inner scoped variable did. This is because the inner variable shadows the outer.