Rust Enum Methods
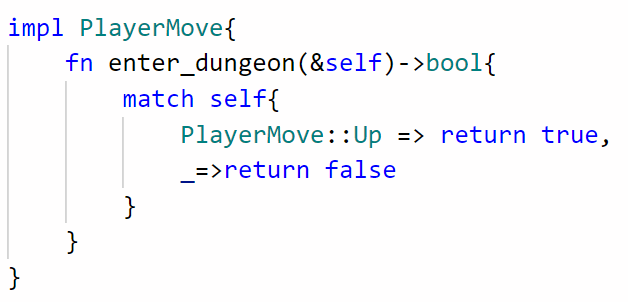
In Rust, enum methods are user defined functions that apply to enums.
Methods are functions that act upon a specific type of object; an enum method is a function that acts on enums.
Enum methods have a similar syntax to struct methods. Like other functions, they are defined using the fn keyword. The first parameter of an enum method is always &self:
fn method_name(&self) {}
In this tutorial, we will cover enum methods in detail.
Enum Method Implementation Block
Once an enum is declared, methods that work on the enum can be defined inside an implementation (impl) block:
impl EnumName {}
Enum methods use the function fn keyword and their first parameter is always &self, which allows the function to act on the enum:
impl EnumName {
fn method_name(&self) {
}
}
Declaring an Enum Method
Enum methods are declared inside the curly braces that define the impl block.
To use an enum method, we need to learn about the &self parameter and .self notation.
&self
As we’ve already seen, the syntax for declaring an enum method differs from other user-defined functions in that the first parameter must be &self.
A method can work with other parameters as well, but they can only come after the &self parameter in the declaration:
fn method_name(&self, param2, param3) {}
If the method will change the instance it is called on, then will instead need to use &mut self as the parameter instead of &self:
fn method_name(&mut self, param2, param3) {}
Using an Enum Method
Enum methods use dot notation to indicate the enum instance and method to be called with the instance:
enum_instance.method_name();
let enum_instance = EnumName::Variant;
enum_instance.method_name();
Let’s combine this with our previous code to see how enum methods are implemented, from start to finish. This example is just a reference to provide syntax clarity, and will be followed by a practical example:
// Declare an enum:
enum EnumName {
Variant1,
Variant2,
}
// Define a method:
impl EnumName {
fn method_name(&self) {}
}
fn main() {
// Create an instance of EnumName:
let enum_instance = EnumName::Variant;
// Call the method on the instance:
enum_instance.method_name();
}
Note that the instance is created and the method is called from inside the main() function.
Now let’s see a practical example of using an enum method. In the following example, we are evaluating a move to determine what type of move it is. If the move is Up, then the player will enter a dungeon.
#![allow(dead_code)]
#[derive(Debug)]
enum PlayerMove{
Up, Down, Left,Right
}
impl PlayerMove{
// If player moves up, enter the dungeon
fn enter_dungeon(&self)->bool{
match self{
PlayerMove::Up => return true,
_=>return false
}
}
}
fn main(){
let up_move = PlayerMove::Up;
println!("Player is moving {:?}", up_move);
println!("Entering the dungeon - {}", up_move.enter_dungeon());
}
Standard Output:
Player is moving Up
Entering the dungeon - true
Note that we are using a match expression to accomplish this. Match expressions are frequently used with enum methods because they can evaluate which variant is being used and execute code based on the variant.
In this case, the match expression evaluates if the variant is ‘Up’. If this is true, then the player will enter the dungeon.