Rust Break Statement
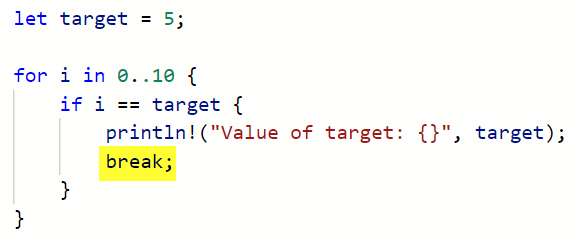
In the Rust programming language, the break statement is used to terminate a loop.
The break keyword is used when you want to stop a loop completely. If you want to restart the loop, use the continue statement instead.
Break is often placed within a conditional expression so that the loop terminates when a specific condition is met.
Break statements are very common, and can be used in all kinds of loops: for, loop, and while. We will cover examples for each type of loop below:
Using break in a for loop
The break statement can be used in a for loop. The following example uses a for loop to determine the value of a target:
let target = 5;
for i in 0..10 {
if i == target {
println!("Value of target: {}", target);
break;
}
}
Standard Output:
Value of target: 5
This is a simple brute-forcing script and in principle is similar to many scanning and brute-forcing tools used in offensive cybersecurity.
The break keyword is used here to exit the for loop when the value of the target has been identified.
Using break with a loop
Using the loop keyword creates an infinite loop, so a break statement is essential to prevent it from running endlessly.
let target = 5;
let mut i = 0;
loop {
i += 1;
if i == target {
println!("Value of target: {}", target);
break;
}
}
Standard Output:
Value of target: 5
This is similar to the previous example but there is a major difference. The loop will operate forever until it finds the target. In contrast, the for loop will only iterate until it reaches the maximum value specified when declaring the for loop.
Note that break is required here; without it, the loop would continue to run endlessly even after it identified the target.
Using break in a while loop
By design, the while loop will only execute when the specified condition is true. Inside the while loop, the break statement adds additional functionality to break the loop using another conditional statement.
In the following example, we are using a while loop to limit our scan to 255. Within the loop, we use the break statement to exit the while loop when the target is identified.
let target = 5;
let mut i = 0;
while i <= 255 {
println!("Loop index: {}", i);
i += 1;
if i == target {
println!("Value of target: {}", i);
break;
}
}
Standard Output:
Loop index: 0
Loop index: 1
Loop index: 2
Loop index: 3
Loop index: 4
Value of target: 5
Based on the output, we can see that the while loop runs 5 times. On the fifth loop, the index i is equal to the target value, so the if expression executes. After the value of the target is printed to the console, the while loop is terminated using the break statement.