Rust Option Enum
Rust has a built-in enum from the standard library called Option. Option has two variants: Some(T), and None.
Option is a built-in enum, so we can use it without declaring it first. However it is helpful to understand what Option looks like:
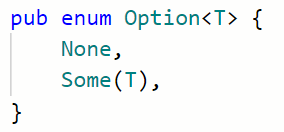
Let’s break this down:
pub – Keyword denoting a public enum, meaning that Option is accessible from external modules.
enum – Keyword specifying an enum; a custom data type with several variants.
Option – The name of the enum.
<T> – Return type of Some<T>. <T> is a type parameter that indicates a generic type. Generics are covered in more detail later in this course.
None – Variant of Option that returns no value.
Some – Variant of Option that returns a value.
The pub keyword indicates that Option is publicly accessible. Following the name ‘Option’ is <T>, which is the type of value returned.
The two variants of Option are None and Some(T), where T indicates the value being returned.
When to Use the Option Enum
The Option enum is easy to use and ideal for a wide range of situations. These include:
- The value of a variable is optional: Cases when we may or may not want a value to be returned.
- Null return values: In general, functions in Rust can’t return a null value because this introduces safety issues. Option can be used to safely return None from a function, which avoids the perils of null values.
- When you may run into an out of bound error: For example if you try to access a vector element using an index greater than the size of a vector minus one.
Using Option When There is No Return Value
The following example shows how to use the Option enum for cases when there is no return value. If the color selected matches “Green” then Option is used to return Some (true). When the wrong color is selected, it returns None.
fn fav_color(color:&str)-> Option<bool> {
if color == "Green" {
Some(true)
} else {
None
}
}
fn main() {
println!("{:?}", fav_color("Green"));
println!("{:?}", fav_color("Blue"));
}
Standard Output:
Some(true)
None
Using Option When Variable Value is Optional
There are often times when we want a variable to have an optional value. For example a user may not input a value an optional value into a requested field.
In the example below, we are building a simple game with two characters. The first uses a turn to move left but the second player decides not to move at all. This is possible using the Option enum. Note that when there is a value, we wrap it in inside the Some() parentheses; when it is None, we just write ‘None’:
#![allow(dead_code)]
#[derive(Debug)]
enum PlayerMove {
Left, Right,
}
#[derive(Debug)]
struct Player {
name: String,
level: i32,
turn: Option<PlayerMove>,
}
fn main() {
let player1 = Player {
name: String::from("Batman"),
level:10,
turn: Some(PlayerMove::Left),
};
let player2 = Player {
name: String::from("Dumbledore"),
level:12,
turn: None,
};
println!("{:?}", player1);
println!("{:?}", player2);
}
Standard Output:
Player { name: "Batman", level: 10, turn: Some(Left) }
Player { name: "Dumbledore", level: 12, turn: None }