Rust for Loop
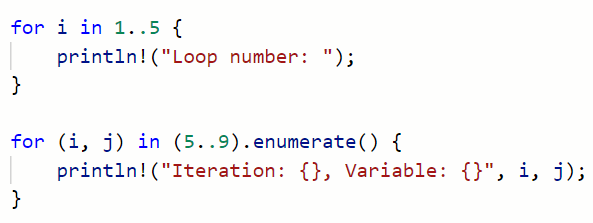
In Rust, the for keyword is used to create a definite loop. As a definite loop, the number of iterations that the for loop performs is known at compile time.
A for loop is defined using the keyword for, followed by a variable and a range:
for <variable> in <range> {}
For example:
for i in 1..5 {
println!("Loop number: {}", i);
}
Standard Output:
Loop number: 1
Loop number: 2
Loop number: 3
Loop number: 4
The for Loop in Rust
The for loop is one of a few types of loops in Rust, which also features indefinite loops like loop and while.
for Loop Range
Unlike other types of loops, a for loop is designed to iterate for a specified number of times, over a designated range.
The range is defined using range notation:
Range notation: a..b
The for loop will loop from the number ‘a’ to the number ‘b’, exclusive of ‘b’.
For example, the range 0..100 will loop from number 0 through number 99. The code inside the block will execute a total of 100 times.
for Loop Variable
The range is paired with a variable; inside the loop, the variable takes the value of each number specified by the range. In the following example, the variable i takes on the values of 1 through 5 within the code block:
for i in 1..5 {
println!("Variable i equals: {}", i);
}
Standard Output:
Variable i equals: 1
Variable i equals: 2
Variable i equals: 3
Variable i equals: 4
The for loop has a code block defined by curly braces ‘{}’.
We can see from the above example that the code inside the block is executed a set number of times defined by the range, which the variable iterates over.
There is no need to increment the variable because the for loop increments it automatically during the next iteration.
The enumerate() Function
The enumerate() function can be used to determine how many times the for loop has iterated.
Using enumerate() requires a slightly more complex syntax:
for (<iteration>, <variable>) in <range>.enumerate() {}
For example:
for (i, j) in (5..9).enumerate() {
println!("Iteration: {}, Variable: {}", i, j);
}
Standard Output:
Iteration: 0, Variable: 5
Iteration: 1, Variable: 6
Iteration: 2, Variable: 7
Iteration: 3, Variable: 8
The above example used two variables named i and j to iterate over. The variable i represents the iteration count, which starts at 0. The variable j is now the variable whose value is determined by the range and the iteration number.
enumerate() provides additional functionality by incrementing both variables simultaneously, and we are free to use them both inside the code block.