Rust Modules
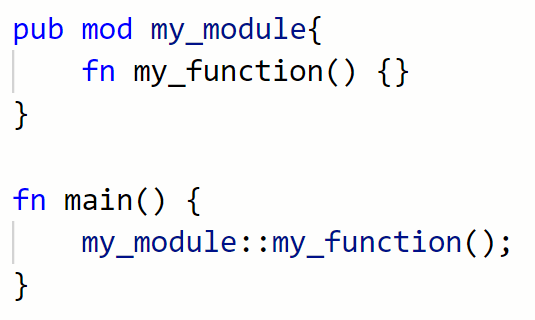
In Rust, a module is a partitioned collection of items that allows developers to efficiently organize code. Modules provide a great deal of control over the scope and privacy of a section of code within a larger project, and are the lowest level of organization within the module system.
Modules can include things like functions, structs, enums, vectors, and arrays. You can think of a module as a box that code can be stored in. The contents of a single module can be public or private, and multiple modules can be nested to create a hierarchical structure of modules.
Modules give developers a great deal of control over how to organize code, and this helps to improve efficiency as well as safety.
In this article, we will cover Rust modules including how to declare modules, how to make a module public using the pub keyword, and how to invoke modules.
Declaring a Module
A module can be declared using the mod keyword.
mod module_name {}
Everything inside the curly braces ‘{}’ is considered part of the module.
Module Naming Convention: By convention, modules are named using snake_case. This means that all letters are lowercase and words are separated with an underscore ‘_’.
Making a Module Public Using pub
Modules are private by default. This means that the code within the module can’t be accessed from outside. This can provide security in situations when we don’t require access to the module’s code from the outside, but it also limits that module’s functionality.
We can make a module public using the pub keyword in the module declaration:
pub mod module_name {}
This will make the entire module public. However, Rust also provides us with fine control over code within a module.
Invoking a Module
A module can be invoked using the scope resolution operator ‘::’.
To invoke a module, we use the following syntax:
module_name::foo;
In this example, ‘foo’ can be a function, struct, array, or another type of data structure that lives inside module_name.
Working With Modules
Let’s see a simple example of how to work with a module. In the following code, we create a public module called my_module, which contains a single function my_function(). Then we call my_function from the main() function.
// Declaring the public module my_module:
pub mod my_module{
// my_function() lives inside my_module
fn my_function() {}
}
fn main() {
// Calling my_function() from outside my_module:
my_module::my_function();
}