Nesting Modules in Rust
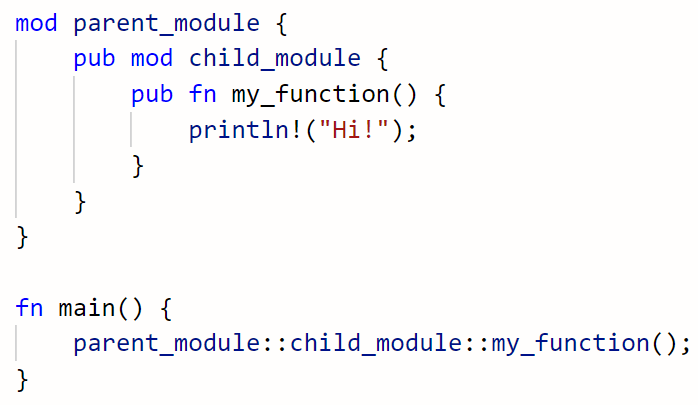
In the Rust programming language, modules can be nested inside each other. This allows developers flexibility in structuring code to allow for maximum utility and safety.
Nesting refers to a structure in which one module lives inside another. Rust gives developers freedom in how we nest modules; any number of modules can be nested in a module, with infinite possible configurations.
A module that contains other modules is called a parent module; the modules it contains are called child modules.
How to Nest Modules
Modules are nested by declaring them inside another module using the mod keyword:
mod parent_module {
mod child_module {}
}
Several child modules can be nested inside a parent module:
mod parent_module {
mod child_module1 {}
mod child_module2 {}
}
Each child module can also be a parent to its’ own child modules, creating a more complex hierarchy of modules:
mod outer_module {
mod inner_module1 {
mod innermost_module1 {}
}
mod inner_module2 {
mod innermost_module2 {}
}
}
Accessing a Nested Module
Similar to invoking a function from a module, nested modules can be accessed using the scope resolution operator ‘::’.
To access a nested module, we use the following sytax:
parent_module::child_module::foo;
Where ‘foo’ is a function, struct, enum, or other object or data structure that lives inside the child_module.
Making Nested Modules Public Using pub
The standard rules for privacy apply when modules are nested inside each other. This means that if we want to access a function that lives inside a child module, both the function and the child module need to be declared public using the pub keyword.
Let’s see an example of this:
mod parent_module {
pub mod child_module {
pub fn my_function() {
println!("Hi!");
}
}
}
fn main() {
parent_module::child_module::my_function();
}
Standard Output:
Hi!
In this example, my_function() is a function that lives inside child_module, which itself lives inside parent_module. We are able to call my_function() from main() by accessing it via its’ parent modules.
For more information on this topic, see our tutorial on functions in modules.