Rust Language Comments
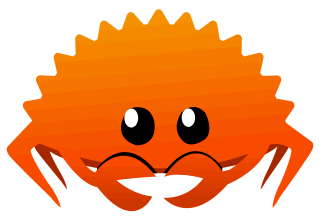
Rust supports both single line and multi-line commenting. Comments can be used to explain Rust code and make it more readable, as well as to enable faster programming and debugging.
Rust uses two forward slashes // to indicate a single line comment and a slash asterisk /* */ to indicate a multi-line comment.
An entire line can be commented out, or the end of a line can be commented out using //.

Creating a Comment in Rust
Comments can be created using two forward slashes ‘//’:
// This is a comment in Rust
The double slash tells Rust to ignore the rest of the line.
We can use comments to prevent code from executing. This means that we don’t have to delete code even if we don’t want it to execute. This is a very useful feature, as it allows us to comment out code that we don’t want to run but might still be useful to us.
fn main() {
// This is a comment
println!("Hello, world!");
}
Comments can be inside or outside function bodies:
// This comment is outside the function body
fn main() {
// This comment is inside the function body
println!("Hello, world!");
}
Multi Line Comments in Rust
Multi-line comments use a slash-asterisk to indicate the start of a comment and asterisk-slash to indicate the end:
fn main() {
/* This is a
multi-line
comment
*/
}
End of Line Comments in Rust
Comments can be placed at the end of the line of code. The code before the comment will still run, but anything after the double slashes will be ignored:
fn main() {
println!("Hello, world!"); // This comment is at the end of a line of code.
}