Rust Arithmetic Operators
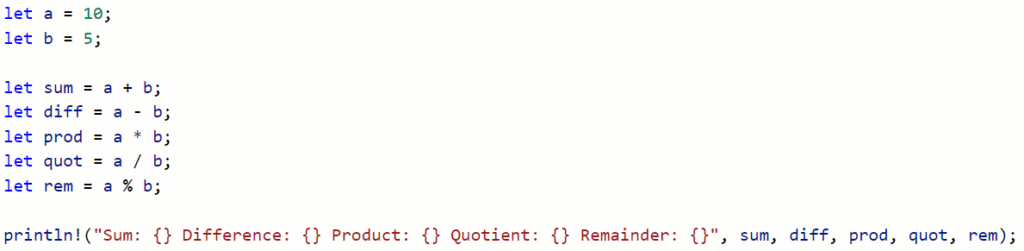
In the Rust programming language, arithmetic operators are symbols that are used to perform mathematical operations.
Arithmetic operators are a type of binary operator because they act on two operands.
There are five basic arithmetic operations in Rust: addition, subtraction, multiplication, division, and remainder.
Each operation has a corresponding operator, as shown in the table below:
Operator | Operation | Syntax | Function |
+ | Addition | a + b | Sum of a and b |
– | Subtraction | a – b | Difference of a and b |
* | Multiplication | a * b | Product of a and b |
/ | Division | a / b | Quotient of a and b |
% | Remainder | a % b | Remainder of division |
In addition to basic arithmetic operators, Rust also has a compound assignment operator for each mathematical operation.
Types of Arithmetic Operators in Rust
There are five basic types of arithmetic operators in Rust: addition, subtraction, multiplication, division, and remainder.
Addition Operator
The addition operator is ‘+‘. It calculates the sum of two values:
let a = 10;
let b = 5;
let sum = a + b;
println!("Sum: {}", sum);
Standard Output:
15
Subtraction Operator
The subtraction operator is ‘-‘. It calculates the difference between two values:
let a = 10;
let b = 5;
let diff = a - b;
println!("Difference: {}", diff);
Standard Output:
5
Multiplication Operator
The multiplication operator is ‘*‘. It calculates the product of two values:
let a = 10;
let b = 5;
let prod = a * b;
println!("Product: {}", prod);
Standard Output:
50
Division Operator
The division operator is ‘/‘. It calculates the quotient of two values:
let a = 10;
let b = 5;
let quotient= a / b;
println!("Quotient: {}", quotient);
Standard Output:
2
Remainder Operator
The remainder operator is ‘%‘ and is also commonly called ‘modulo‘. It calculates the remainder of division between two values:
let a = 11;
let b = 5;
let mod= a % b;
println!("Remainder: {}", mod);
Standard Output:
1
In this example the remainder operator produced an output of 1 because 11 divided by 5 is 2, with a remainder of 1.
Compound Assignment Operators
In addition, Rust allows us to perform an arithmetic operation with assignment simultaneously. These are also known as compound assignment operators.
There are five compound assignment operators, one for each arithmetic operator:
- Addition and assignment: +=
- Subtraction and assignment: -=
- Multiplication and assignment: *=
- Division and assignment: /=
- Remainder and assignment: %=
Addition and Assignment Operator
The addition and assignment operator is ‘+=‘. It will add the value from the right side of the assignment operator ‘=’ to the variable on the left side, and assign the sum to the variable on the left.
let mut a = 5;
a += 3;
println!("{}", a);
Standard Output:
8
Subtraction and Assignment Operator
The subtraction and assignment operator is ‘-=‘. It will subtract the value from the right side of the assignment operator ‘=’ from the variable on the left side, and assign the difference to the variable on the left.
let mut a = 5;
a -= 3;
println!("{}", a);
Standard Output:
2
Multiplication and Assignment Operator
The multiplication and assignment operator is ‘*=‘. It will multiply the value from the right side of the assignment operator ‘=’ and the value of the variable on the left side, and assign the product to the variable on the left.
let mut a = 5;
a *= 3;
println!("{}", a);
Standard Output:
15
Division and Assignment Operator
The multiplication and assignment operator is ‘/=‘. It will divide the value from the left side of the assignment operator ‘=’ by the value of the variable on the right side, and assign the quotient to the variable on the left.
let mut a = 5;
a /= 3;
println!("{}", a);
Standard Output:
1
Note that division can be more complex than other arithmetic operations because we can’t cleanly divide most integers without a remainder and we can’t divide by 0. See the article on Numbers in Rust for more details.
Remainder and Assignment Operator
The remainder and assignment operator is ‘%=‘. It will divide the value from the left side of the assignment operator ‘=’ by the value of the variable on the right side, and assign the remainder of the division to the variable on the left.
let mut a = 5;
a /= 3;
println!("{}", a);
Standard Output:
2
Limitations of Arithmetic Operators in Rust
In Rust, arithmetic operators are designed to function with numerical types like integers or floats.
For example, Rust doesn’t allow us to concatenate two strings with the addition operator ‘+’.
Similarly, we can’t multiply a string using ‘*’.
These are common features in modern programming languages but Rust encourages greater type safety and will throw an error in these cases.