Rust Comparison Operators
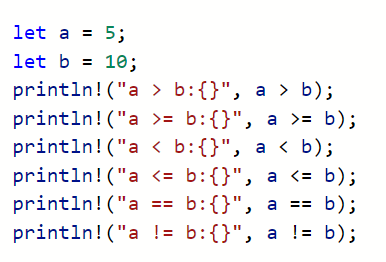
In Rust, comparison operators are used to compare the value of two operands and output a Boolean value.
Comparison operators include greater than, lesser than, equal to, greater than or equal to, lesser than or equal to, and not equal to. These operators provide a good amount of flexibility when working with compatible types.
Comparison operators function differently depending on the type of the operands. The most common use case is using comparison operators with numerical types. However they can also be used with Boolean types and function as a kind of logic gate.
Table of Comparison Operators in Rust
Operator | Operation | Syntax | Function |
> | Greater than | a > b | True if a is greater than b |
< | Lesser than | a < b | True if a is less than b |
== | Equal to | a == b | True if a is equal to b |
>= | Greater than or equal to | a >= b | True if a is greater than or equal to b |
<= | Lesser than or equal to | a <= b | True if a is less than or equal to b |
!= | Not equal to | a != b | True if a is not equal to b |
Using Comparison Operators
Comparison operators in Rust use a simple, intuitive syntax.
The following code example shows how each comparison operator can be used:
let a = 5;
let b = 10;
println!("a > b:{}", a > b); // false
println!("a >= b:{}", a >= b); // false
println!("a < b:{}", a < b); // true
println!("a <= b:{}", a <= b); // true
println!("a == b:{}", a == b); // false
println!("a != b:{}", a != b); // true
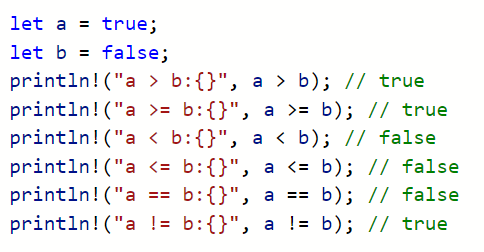
Using Comparison Operators with Boolean Operands
In Rust, comparison operators can be used with Boolean operands to perform logical functions. As a result, they can be used to extend the functionality provided by logical operators.
When used with Boolean operands, each comparison operator will function by regarding a Boolean value of true as the equivalent numerical value of 1. A Boolean value of false has an equivalent numerical value of 0:
Boolean Value | Numerical Value |
True | 1 |
False | 0 |
The following image shows how comparison operators function with Boolean operands in Rust:
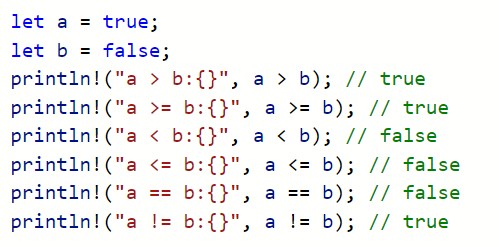
We can construct Truth tables to see how each operator would function as a logic gate.
Greater Than Operator
The greater than operator is true only if A is true and B is false.
Operand A | Operand B | A > B |
True | True | False |
True | False | True |
False | True | False |
False | False | False |
Lesser Than Operator
The lesser than operator is true only if A is false and B is true.
Operand A | Operand B | A < B |
True | True | False |
True | False | False |
False | True | True |
False | False | False |
Equal To Operator
The lesser than operator is true if A and B are either both true or false.
Operand A | Operand B | A == B |
True | True | True |
True | False | False |
False | True | False |
False | False | True |
Greater Than or Equal To Operator
The greater than or equal to operator is false only if A is false and B is true.
Operand A | Operand B | A >= B |
True | True | True |
True | False | True |
False | True | False |
False | False | True |
Lesser Than or Equal To Operator
The greater than or equal to operator is false only if A is true and B is false.
Operand A | Operand B | A <= B |
True | True | True |
True | False | False |
False | True | True |
False | False | True |
Not Equal To Operator
The not equal to operator is true if A is not equal to B.
Operand A | Operand B | A <= B |
True | True | False |
True | False | True |
False | True | True |
False | False | False |