Rust if let
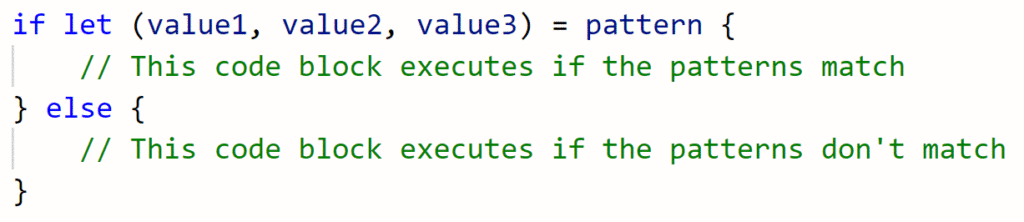
In Rust, if let is a conditional expression that allows pattern matching within an if statement. If the pattern matches a scrutinee expression, then a resulting block of code will be executed.
The if let expression doesn’t necessarily add new capability to if statements or match expressions, but it allows us to use a cleaner, simpler syntax in certain cases.
For example, if let is similar to the match expression, but we don’t need to provide code for every possible expression. In addition, if let expressions allow us to use several values within the scrutinee expression, making it ideal for general pattern matching. One example is a situation in which we are comparing one set of values with another set of values.
Another case where if let can be useful is when we want to match part of a pattern rather than the whole thing.
In this tutorial, we will cover if let expressions including syntax, using if let with else expressions, using if let to match patterns as well as values, using if let to match part of a pattern, and the related while let expression.
if let Expression Syntax
Following the if let keywords, we include the value to be matched against on the left side of the ‘=’ operator and the scrutinee expression (to be matched) on the right side of the ‘=’ operator.
if let value1 = value2 {}
The code inside the block (denoted by the curly braces {}) will execute only if value1 is equal to value2.
In the case of comparing more complex patterns such as sets of values, we can place the pattern inside parentheses:
if let (value1, value2, value3) = pattern {}
Using an Else Expression With if let
By itself, if let will only handle the case in which the two patterns match. This can be useful by itself because there are many times when we don’t need to handle cases when two patterns don’t match. In terms of functionality, this is similar to using an if expression without an else expression.
Just like the if expression, we can add an else expression to if let in order to address cases where the two patterns don’t match:
if let (value1, value2, value3) = pattern {
// This code block executes if the patterns match
} else {
// This code block executes if the patterns don't match
}
Let’s see a few examples of if let expressions in action!
Using if let to Match a Single Value
The following simple case shows how we can use an if let expression to match a single string value:
fn main() {
let course = "Rust";
if let "Rust" = course {
println!("The patterns match!");
} else {
println!("The patterns don't match!");
}
}
Standard Output:
The patterns match!
In this case, the patterns match so the code block in the if let statement executes; if the patterns didn’t match then the else block would have executed.
Using if let to Match Patterns
Let’s look at the case of matching a more complex pattern. In the following example, we create a tuple named course that contains two strings. We then use if let to compare the tuple with a tuple pattern that we specify:
fn main() {
let course = ("Rust", "lang");
if let ("Go", "lang") = course {
println!("The patterns match!");
} else {
println!("The patterns don't match!");
}
}
Standard Output:
The patterns don't match!
In this case, the patterns don’t match, so the code inside the else {} block gets executed.
We Don’t Need to Specify All of The Values With if let
Interestingly, Rust allows us to pattern match with if let without specifying all of the values in a pattern. This allows if let to match part of a pattern rather than the whole thing.
Recall that in the previous example, the else {} block executed because the the first element in the tuples didn’t match.
We can resolve this by replacing the first element in the if let expression pattern with a dummy variable:
fn main() {
let course = ("Rust", "lang");
if let (a, "lang") = course {
println!("The patterns match!");
} else {
println!("The patterns don't match!");
}
}
Standard Output:
The patterns match!
This feature significantly extends the applicability of if let expressions.
Rust while let Expressions
Similar to the if let expression, a while let expression can be used when we want to loop over a block of code when a pattern is matched:
fn main() {
let mut my_vector = vec![1, 2, 3, 4, 5];
while let Some(num) = my_vector.pop() {
println!("{}", num);
}
}
Standard Output:
5
4
3
2
1