Rust Variables
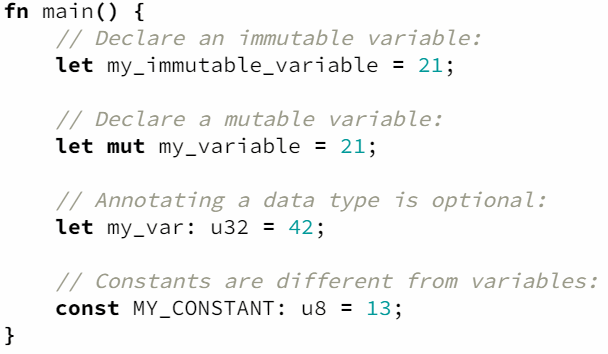
In Rust, a variable is a value that is paired with a name. Variables can be either mutable or immutable, and are immutable by default.
Rust uses the let keyword to declare a variable:
let my_variable = 42;
Let’s break this down:
let is used to declare the variable
my_variable is the name of the variable
42 is the value being assigned to my_variable
Variable Types in Rust
Rust will automatically assign a data type to a variable if one is not specified. Take the previous example:
let my_variable = 42;
Since we set my_variable equal to 42, Rust will use the default integer type, which is i32 – a signed 32 bit integer.
Rust allows us to specify the variable type:
let my_variable: u8 = 42;
In this case, we have assigned a data type of u8 – an unsigned 8 bit integer.
Rust Variable Mutability
Variables are immutable by default but can be made mutable by including the keyword mut in the variable declaration:
let mut my_variable = 42;
This will allow us to change my_variable after an initial value has been assigned:
fn main() {
let mut my_variable = 21;
println!("The value of my_variable is: {}", my_variable);
my_variable = 42;
println!("The value of my_variable is: {}", my_variable);
}
Output:

Declaring Multiple Variables
We can create multiple variables with a single line of code:
let (name,experience) = ("Bill","beginner");
println!("My name is {} and I am a {} in Rust.", name, experience);
Variables vs. Constants in Rust
There are two kinds of name-binding declarations in Rust: variable declarations and constant declarations. Constants are also commonly referred to as constant variables.
There are several differences between variables and constants:
- Variables can be either mutable or immutable (constants are always immutable).
- Variables are declared using let, while constants are declared using const.
- Variables do not require the data type to be specified (constants require data type annotation).
- Variables are constrained to live in a block (between curly braces {}); constants can be global in scope.
- Variables should use snake_case while constants should use SCREAMING_SNAKE_CASE.
Declaration Keyword | Syntax | Type | |
Variables | let | snake_case | Not required |
Constants | const | SCREAMING_SNAKE_CASE | Required |
Naming Convention for Variables in Rust
Carbon uses snake_case for variables. This means that all letters are lowercase, and words are separated using an underscore. The snake_case style should be used for all variables, whether mutable or immutable.
In contrast, constants should always use SCREAMING_SNAKE_CASE. This helps to differentiate variables from constants.
Variable Errors in Rust
Rust has a lot of features to help us improve our code, and many of these features are built-in using errors.
When it comes to variables, Rust will not compile code that contains unnecessary variables or variables that are unnecessarily made mutable. As a result, Rust will throw errors for many variable-related issues including:
- Variables declared but not used
- Mutable variables that are never changed (variables that don’t need to be mutable)
- Immutable variables that are changed (variables that should be mutable)
- Mutable variables that are assigned an unused value (if a mutable value is assigned a value that is unused but later changed)
These errors can help us to streamline our code base and reduce unneeded bloat that can accumulate in larger projects.